
Building a complete digital marketplace with Next.js 14, React, tRPC, and Tailwind CSS is an exciting project that allows you to create a modern, efficient, and visually appealing platform.
Here's a step-by-step guide to help you get started:
Step 1: Setting Up Your Next.js Project
Initialize your Next.js project: npx create-next-app@latest my-marketplace
Navigate to your project directory: cd my-marketplace
Install necessary dependencies: npm install react react-dom swr trpc tailwindcss
Step 2: Setting Up tRPC
Create a .trpc.ts file in your project root:
import { createTRPCClient } from '@trpc/client';
import { AppRouter } from '../api/_trpc';
export const trpc = createTRPCClient<AppRouter>({
url: '/api/trpc',
});
2. Create a api/_trpc.ts file:
import { createTRPCRouter } from '@trpc/server';
import { z } from 'zod';
export const AppRouter = createTRPCRouter()
.query('hello', {
resolve() {
return { text: 'Hello, World!' };
},
})
.mutation('add', {
input: z.number(),
resolve({ input }) {
return input + 1;
},
});
Step 3: Setting Up Tailwind CSS
Configure Tailwind CSS:
npx tailwindcss init -p
2. Create a tailwind.config.js file in your project root:
module.exports = {
mode: 'jit',
purge: ['./pages/**/*.{js,ts,jsx,tsx}', './components/**/*.{js,ts,jsx,tsx}'],
darkMode: false, // or 'media' or 'class'
Step 4: Building Your Marketplace
Create your marketplace pages in the pages directory:
pages/index.tsx: Homepage
pages/products.tsx: Products listing page
pages/product/[id].tsx: Product detail page
pages/cart.tsx: Shopping cart page
pages/checkout.tsx: Checkout page
// Example of pages/index.tsx
import React from 'react';
import Head from 'next/head';
import Header from '../components/Header';
import Footer from '../components/Footer';
import ProductCard from '../components/ProductCard';
import { useQuery } from '../hooks/useQuery';
const Home: React.FC = () => {
const { data: products, error } = useQuery('products');
if (error) return <div>Error loading products</div>;
if (!products) return <div>Loading...</div>;
return (
<div>
<Head>
<title>My Marketplace</title>
<link rel="icon" href="/favicon.ico" />
</Head>
<Header />
<main className="container mx-auto my-8">
<h1 className="text-3xl font-semibold mb-4">Featured Products</h1>
<div className="grid grid-cols-1 sm:grid-cols-2 md:grid-cols-3 lg:grid-cols-4 gap-4">
{products.map((product: any) => (
<ProductCard key={product.id} product={product} />
))}
</div>
</main>
<Footer />
</div>
);
};
export default Home;
2. Create necessary components in the components directory:
components/Header.tsx: Navigation header
components/Footer.tsx: Footer
components/ProductCard.tsx: Card for displaying product information
components/CartItem.tsx: Cart item component
// Example of components/ProductCard.tsx
import React from 'react';
import Link from 'next/link';
const ProductCard: React.FC<{ product: any }> = ({ product }) => {
return (
<Link href={`/product/${product.id}`}>
<a>
<div className="bg-white rounded shadow p-4">
<img src={product.image} alt={product.title} className="w-full h-48 object-cover mb-4" />
<h2 className="text-lg font-semibold">{product.title}</h2>
<p className="text-gray-600">${product.price}</p>
</div>
</a>
</Link>
);
};
export default ProductCard;
3. Implement tRPC hooks for data fetching:
Create hooks directory and add useQuery.ts and useMutation.ts files to handle data fetching and mutations respectively.
// Example of hooks/useQuery.ts
import { trpc } from '../.trpc';
import { useQuery } from 'react-query';
export const useQuery = (key: string) => {
return useQuery(key, () => trpc.products.list());
};
4. Style your components using Tailwind CSS:
Utilize Tailwind CSS classes in your components to style them as desired.
// Example of components/Header.tsx
import React from 'react';
import Link from 'next/link';
const Header: React.FC = () => {
return (
<header className="bg-gray-800 text-white py-4">
<div className="container mx-auto flex justify-between items-center">
<Link href="/">
<a className="text-xl font-semibold">My Marketplace</a>
</Link>
<nav>
<ul className="flex space-x-4">
<li>
<Link href="/products">
<a className="hover:text-gray-300">Products</a>
</Link>
</li>
<li>
<Link href="/cart">
<a className="hover:text-gray-300">Cart</a>
</Link>
</li>
</ul>
</nav>
</div>
</header>
);
};
export default Header;
Step 5: Running Your Project
Start your development server:
npm run dev
2. Navigate to http://localhost:3000 in your browser to view your marketplace.
By following these steps, you'll be well on your way to building a complete digital marketplace using Next.js 14, React, tRPC, and Tailwind CSS. This modern stack provides a powerful foundation for creating efficient, responsive, and visually appealing web applications. Happy coding!
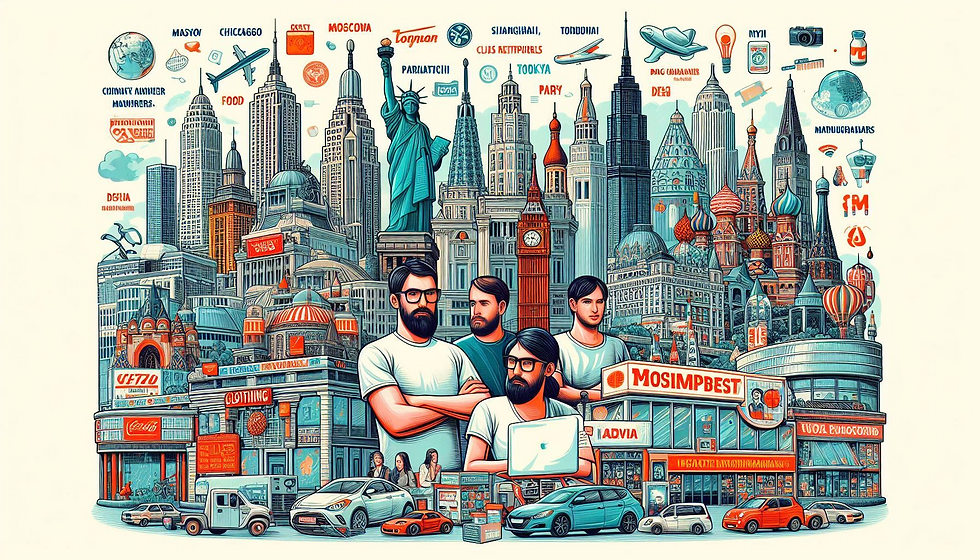
More here:
Welcome
Comments